Microservices - Centralized Configurations With Spring Cloud Config
-
Chinthaka Dinadasa
- 13 Mar, 2022
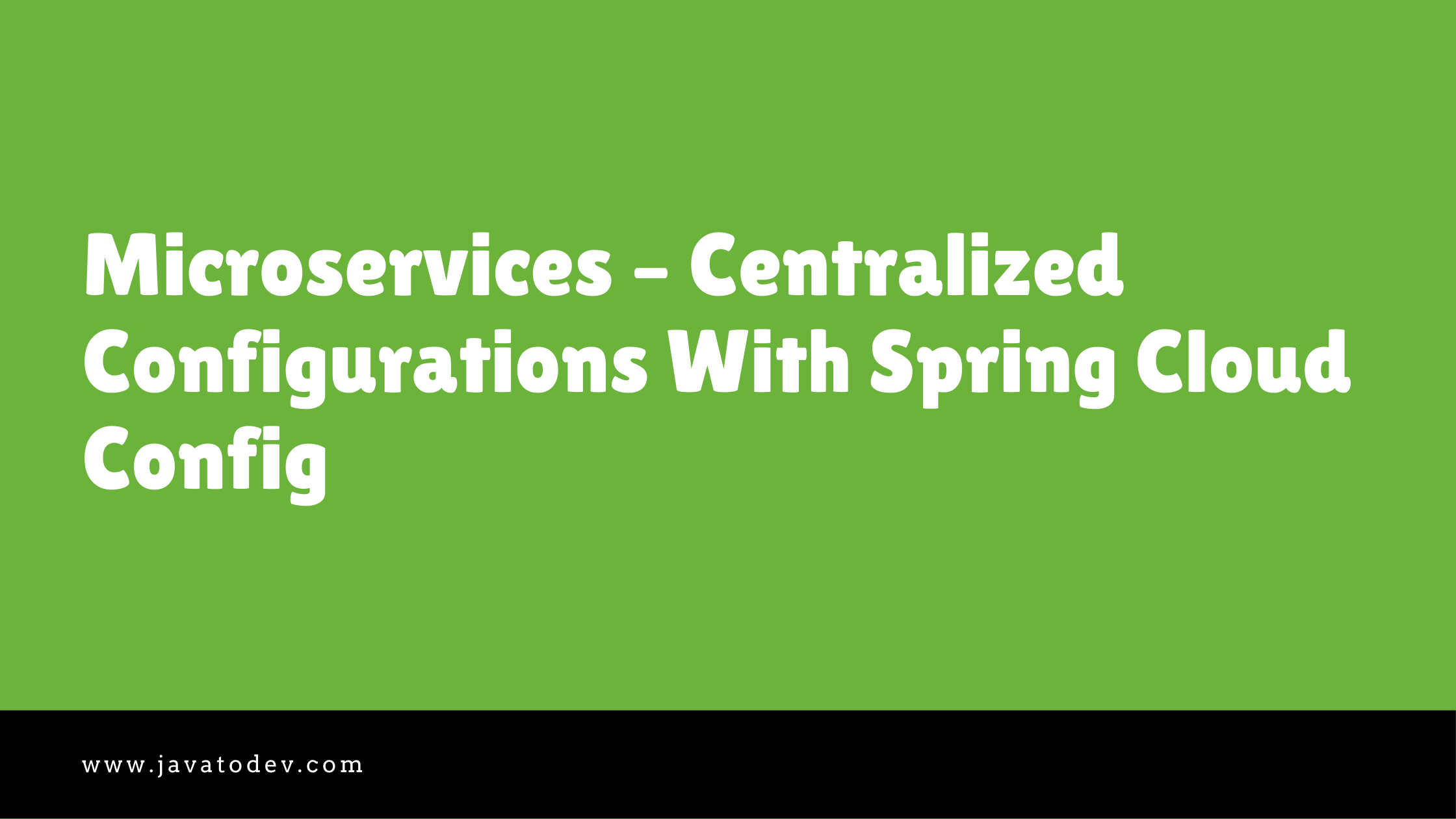
Spring cloud config is provides externalized configurations for a distributed application. I’m going to explain how we can set up a spring cloud configuration server and consume that configuration server with a spring boot microservices project.
Problem and Solution
In microservices architecture there can be hundreds of independent services which has been deployed. Traditionally we are using environment based config or application level configurations. It could hard to manage with the growth of the application architecture. So here we have an approach called centralized configurations where we manage all the application configurations in a central location and all the services inside the architecture will consume that central location to capture all necessary configurations.
In addition to that this could be implemented in a secure way using both application level configs and infrastructure level configurations to minimize unauthorized access to applications configurations.
In this tutorial, I’ll demonstrate how we can set up a spring cloud Configuration Server and configure multiple application properties using GitHub repository, and connect those with microservices inside a spring boot microservice project using Config Client a Client that connects to a configuration server to fetch the application’s configuration.
Centralized Configurations For Microservices Using Spring Cloud Config Server
This is an article from Building Microservices With Spring Boot – Free Course With Practical Project, and you can directly download all the source codes for this tutorial from our GitHub repository. Please use the branch named feature/spring-cloud-config-server-setup to follow these steps in this article.
Setting Up Spring Cloud Config Server
This is the base spring boot project which listens to centralized configurations from a given source (Git) and makes responses to incoming configuration request from client microservices. Additionally, the configuration server could load configuration via Git, SVN, or HashiCorp Vault.
Just create another spring boot application by adding the following dependencies,
Spring cloud config server creation using spring initializr
After that, we need to enable config server capabilities to created a spring boot application by adding @EnableConfigServer in the configuration class or in the application main class.
package com.javatodev.finance;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.config.server.EnableConfigServer;
@EnableConfigServer
@SpringBootApplication
public class InternetBankingConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(InternetBankingConfigServerApplication.class, args);
}
}
Now there is a requirement of having a Git, SVN, or Hashicorp vault to store our application configurations which we need to configure with this configurations server.
Let’s create a repository and add application configurations of all the microservices inside our architecture. Additionally, this repository could be a private repository or a public repository. I’ll include all the necessary steps to access the private repository with this article.
Here in this GitHub repository, I’ve created the same application configurations that I’ve configured in multiple microservices in our architecture. You can refer to that public repository or create your own set of configurations with a repository. We just need the repo URL and credentials to access it when it is a private repository.
Few Tips For Creating Centralized Application Configurations
-
You can choose both .yml or .properties extensions when storing application configurations in spring cloud server.
-
If you need to create property files for multiple spring boot profiles (DEV/STAGING/PRODUCTION), just create separate configrations file as follows, Here spring cloud config capture profile with value after – inside file name.
- internet_banking_api_gateway.yml – Default application yml
- internet_banking_api_gateway-dev.yml – Application YML for DEV profile
- internet_banking_api_gateway-staging.yml – Application YML for STAGING profile
- internet_banking_api_gateway-prod.yml – Application YML for PRODUCTION profile
More Configurations That Connects Github
Now since we have a configuration server and already defined app configurations in a sharable location, we can focus on configuring this with the spring cloud config server.
Just add the following configurations into the application.yml or application.properties file in spring-cloud-config enabled spring boot application that we have created earlier.
server:
port: 8090
spring:
cloud:
config:
server:
git:
uri: https://github.com/javatodev/internet-banking-configurations.git
search-paths: configuration
default-label: main
- spring.cloud.config.server.git.uri – This is the URL to access github repository which keeps all our application configurations.
- spring.cloud.config.server.git.search-paths – Here I’m setting whatever the folder structure that we are keeping where I’ve stored all the configuration in github repository. (Optional)
- default-label – Branch that configurations server should check for configurations.
- In addition to that if you are using a private repository just use spring.cloud.config.server.git.username and spring.cloud.config.server.git.password to set authentication.
After configuring all the things just start the service as a spring boot application. Then you can check all the things by accessing the following URLs.
- http://localhost:8090/internet\_banking\_api\_gateway/default – This loads all the configurations inside default property file of internet-banking-api-gateway microservice.
Internet Banking API Gateway Configurations Load Through Spring Cloud Config Server
- http://localhost:8090/internet\_banking\_api\_gateway/dev – This will return all the configuraration under DEV profile along with default profile. In spring boot it will automatically give priority to DEV profile, when run with DEV profile.
Internet Banking API Gateway Configurations Load Through Spring Cloud Config Server
Now we have a working configuration server, Then let’s focus on configuring microservices to use configuration server in order to load application configurations.
Configure Microservices with Config Client
Let’s focus on how to set up microservices inside our architecture to consume the configurations server and capture all the application properties when deploying the application.
Here I’ll demonstrate the approach with internet-banking-api-gateway and the process is the same for every and each other service inside the architecture.
First, we should include dependencies that should be present on configuration server consumers,
//SPRING CLOUD CONFIG
implementation 'org.springframework.cloud:spring-cloud-starter-config'
implementation 'org.springframework.cloud:spring-cloud-starter-bootstrap'
After that create bootstrap.yml inside src/main/resources and add the following configurations which this application connect with the configuration server which we have configured earlier.
spring:
cloud:
config:
uri: http://localhost:8090
Finally, remove all the local configurations from application.yml or application.properties and keep spring.application.name configuration only, This value will be used by the spring cloud config client and resolve configurations using the configuration server.
spring:
application:
name: internet_banking_api_gateway
All done now you just need to start both configuration server and API gateway which we have configured.
Then you can see the following log when running API-gateway,
ConfigServicePropertySourceLocator : Fetching config from server at : http://localhost:8090
ConfigServicePropertySourceLocator : Located environment: name=internet_banking_api_gateway, profiles=[default], label=null, version=0c584208a77e36ac64803e6d22160e4ce6397426, state=null
PropertySourceBootstrapConfiguration : Located property source: [BootstrapPropertySource {name='bootstrapProperties-configClient'}, BootstrapPropertySource {name='bootstrapProperties-https://github.com/javatodev/internet-banking-configurations.git/configuration/internet_banking_api_gateway.yml'}]
InternetBankingApiGatewayApplication : No active profile set, falling back to default profiles: default
All done and API gateway should start and respond to the requests now,
Conclusion
Thanks for reading our latest article on Microservices – Centralized Configurations With Spring Cloud Config with practical usage.
If you are looking for spring boot practical application development tutorials, just check our article series.
You can get the source code for this tutorial from our GitHub repository.