Building Microservices With Spring Boot - Free Course With Practical Project
-
Chinthaka Dinadasa
- 06 May, 2021
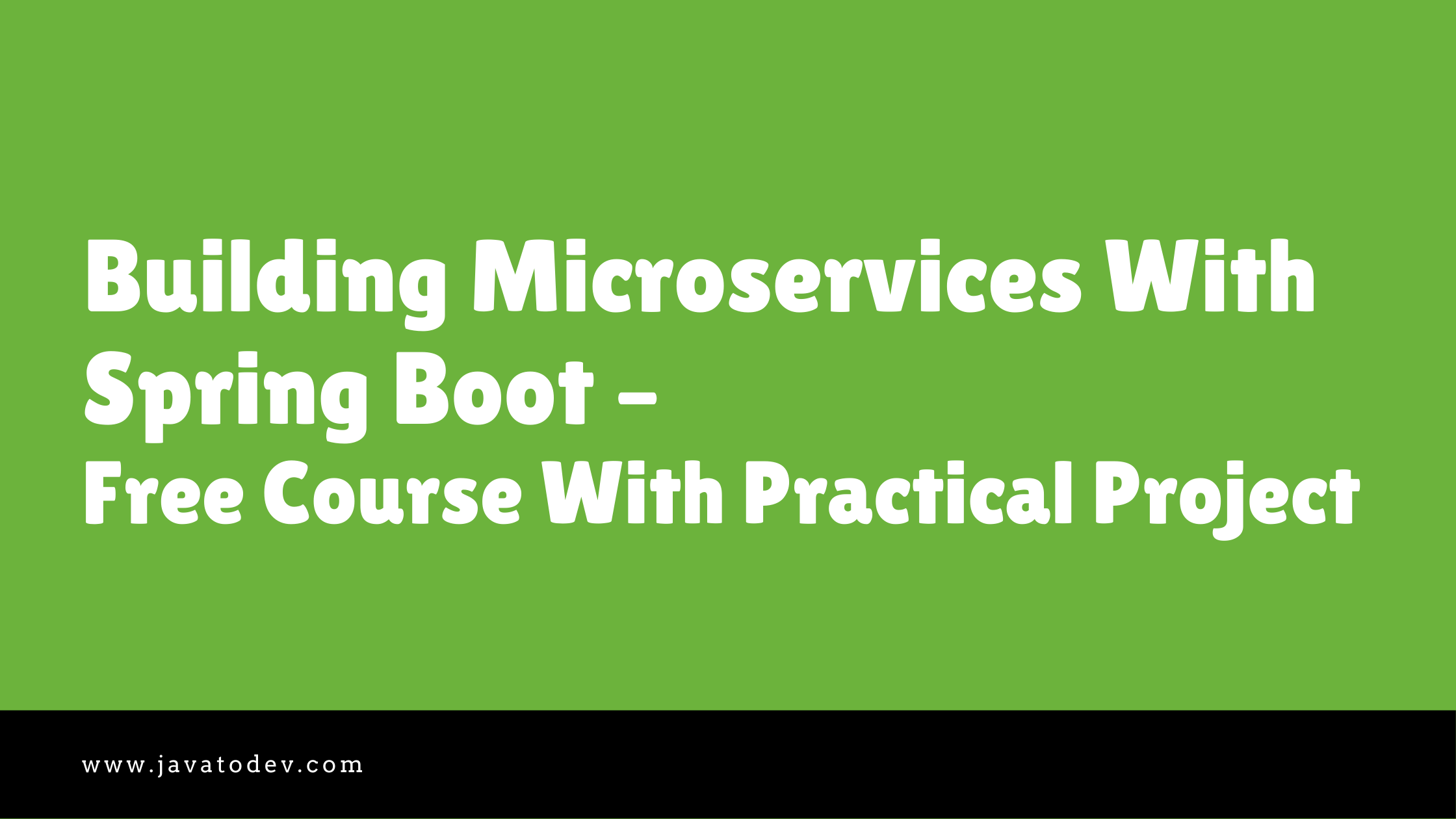
Hello readers, Here I’m starting my new article series on building Microservices with Spring Boot, and I’m going to write this as a free course on practical microservices building using Spring Boot.
There are so many examples on the internet if you have checked on google about how we can build microservices architecture using spring boot and other related technologies. But there are really fewer examples of building proper microservices architecture from scratch to deployments and monitoring.
Hence from here, this article series will include a practical project and how we can initiate our work, and how we can integrate awesome components into our microservices architecture.
Introduction
In this article series I’m going to explain using internet banking API concept with spring boot based microserices architecture. Initially I’ll develop the core API which will evolve as a full fledged REST API collection until deployments.
Microservices
Here this project consist of mainly 5 microservices and those are,
-
User service (banking-core-user-service) – This service includes all the operations under the User such as registrations and retrieval. Additionally, this API consumes keycloak REST API to register and manage the user base while using the local PostgreSQL database as well.
-
Fund transfer service (banking-core-fund-transfer-service) – This is the service that handles all the fund transfers between accounts and this API will push messages to a centralized RabbitMQ queue to use from the Notification service.
-
Payment service (banking-core-payments-service) – This service will include all the API endpoints to process Utility payments in this project and that will push notification messages to RabbitMQ as well.
-
Notification service – This API is registered under the service registry but consumes all the messages from RabbitMQ and pushes necessary notifications to the end users.
-
Banking core service – This is the banking core service that acts as a dummy banking core with accounts, users, transaction details, and processors for banking transactions.
Microservices Architecture For Internet Banking Concept
Other components inside this architecture
- Service Registry – For this, I’m going to use Netflix Eureka Service Registry.
- API Gateway – Gateway will be configured using Spring cloud gateway.
- Configuration Service – This will be deployed using Spring Cloud Config Server and Client.
- Authentication – Here I’m using Keycloak for authentication and authorization, Keycloak is a open-source Identity management system and we could easily build user related stuff. Additionally, keycloak comes with a handy REST API that allows us to communicate as an API and get things done within seconds.
- Database – For this tutorial will use multiple MySQL database instances.
- Message Queues – RabbitMQ will be the main message queue supplier in this project and I’m having an idea of integrating more tools like RabbitMQ into this same project in the future.
- Logging – ELK stack will be used to manage logs and process custom datasets for quick queries inside the architecture.
- Server API Documentation – API documentation for microservices will be done using Swagger with UI.
- Monitoring – Here we are using Prometheus, Micrometer, and Grafana to monitor the whole application setup after deploying into the servers.
- Distributed tracing – Zipkin will be the tool that we are going to use with this project in order to trace whole application requests and details about the performance.
From here I’ll explain this whole project with multiple blog articles and will share sources over GitHub.
Article Series
Pending for the moment, Let’s meet with our first article soon.
- Microservices – Service Registration and Discovery With Spring Cloud Netflix Eureka
- Microservices – Setup API Gateway Using Spring Cloud Gateway
- Microservices – Authentication, and Authorization With Keycloak
- Microservices – Core Banking Service Implementation
- Microservices – User Service Implementation
- Microservices – Fund Transfer Service Implementation
- Microservices – Utility Payment Service Implementation
- Microservices - Communication With Spring Cloud OpenFeign
- Microservices - Exception Handling
- Microservices - Centralized Configurations With Spring Cloud Config
Conclusion
Thanks for reading our initial article for Building Microservice with Spring Boot article series.
If you are looking for practical spring boot application development tutorials, just check our article series and comment on whatever new things you need to see on our website.
You can get the source code for this tutorial from our GitHub repository.